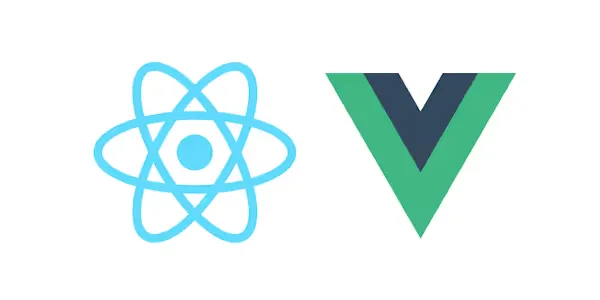
目次
はじめに
ReactとVueはどちらも人気のJavaScriptライブラ/リフレームワークです。
それぞれメリット・デメリットがあり、フロントエンドの技術選定の際にどちらを採用するか悩むことも多いと思います。
この記事では、Reactの公式チュートリアルである「三目並べ」をVueでも書いてみて、ReactとVueのコードを比較してみます。
Reactの公式チュートリアルはこちらです。
チュートリアル:三目並べ – React
The library for web and native user interfaces
https://ja.react.dev/learn/tutorial-tic-tac-toe
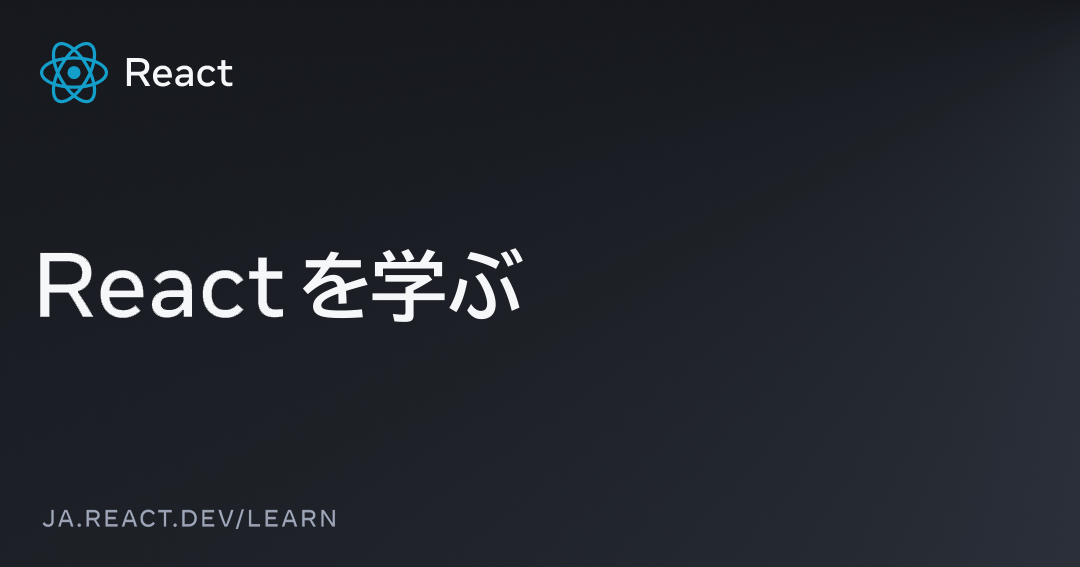
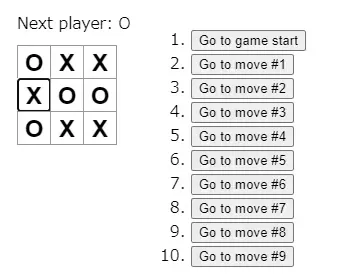
Reactの公式チュートリアル 三目並べ
作成したコードについて
チュートリアル内ではJavaScriptは単一ファイルになっていますが、今回は比較しやすくするためにReactもVueもコンポーネントごとにファイルを分けます。
ReactはCodeSandbox、VueはVue SFC Playgroundにコードを置いているので、すぐに動作確認できます。
バージョン
コーディング時のバージョンは以下の通りです。
フレームワーク | バージョン |
---|---|
react | 18.3 |
Vue | 3.4 |
Reactのコード
Vueのコード
コード比較
コンポーネントごとにコードを比較してみます。
App.js/App.vue
App.js/App.vueは、エントリーファイルとなるコンポーネントです。
ゲーム全体で使用する変数もここで定義しています。
Reactコード
Vueコード
ReactでuseState
を使用している変数は、Vueではref
を使用して定義しています。
また、計算して算出される値はVueではcomputed
を使用して定義しています。
HTMLの記述はReactではJSX、VueではHTMLベースのテンプレート構文になっています。
Board.js/Board.vue
Board.js/Board.vueは、三目並べの盤面を定義するコンポーネントです。
Reactコード
Vueコード
コンポーネントの引数は、ReactではJavaScriptの関数と同じように記載できますが、VueはdefineProps
とdefineEmits
という構文で記載する必要があります。
Square.js/Square.vue
Square.js/Square.vueは、三目並べの盤面内のそれぞれのマスを定義するコンポーネントです。
Reactコード
Vueコード
こちらもコンポーネントの引数の定義方法に差があります。
Moves.js/Moves.vue
Moves.js/Moves.vueは、ゲーム履歴の表示と履歴へ遷移するためのボタンを表示するコンポーネントです。
Reactコード
Vueコード
Reactは任意の位置でJSXを記載できるため、簡潔なコードになっています。
Moves.js/Moves.vue
内のボタンをクリックすると、App.js/App.vue
のcurrentMove
の値が変更されて、過去の手番に戻ります。
上記のコードではどちらも親コンポーネントの関数を子コンポーネントで実行することによって親コンポーネントの変数を変更しています。
Vueの双方向バインディングについて
Vueの場合は双方向バインディングが可能なため、別の記載方法も考えられます。v-model
とdefineModel
を使用すると子コンポーネント側で親コンポーネント側の変数を直接変更できます。
以下がコードの例です。
双方向バインディングはメリット・デメリットがあるため、実際に使うかは検討が必要です。
React/Vueのコードの違いを考察
ReactとVueのコードを比較して分かった両者の特徴を記載してみます。
React
Reactの特徴
- コンポーネントの引数は関数の引数としてシンプルに記述できる
- JavaScript内の任意の箇所でHTML(JSX)を記載できる
Vue
Vueの特徴
- JavaScriptとHTMLの境界が分かりやすい
- HTMLのテンプレート構文がHTMLに似ているため理解しやすい
コードを比較してみると、ReactはJavaScriptが軸となっており、VueはHTMLを軸としている印象を受けました。
プロジェクトやメンバーの技術スタックが、JavaScriptとHTMLどちらに寄っているかも選定の基準の一つとするのもありかもしれません。
まとめ
この記事では、Reactの公式チュートリアルである「三目並べ」をVueでも書いてみて、ReactとVueのコードを比較しました。
ReactもVueもメリットがあるため、プロジェクトやメンバーに応じて選定を行うのが良さそうです。
参考になれば幸いです。
関連記事
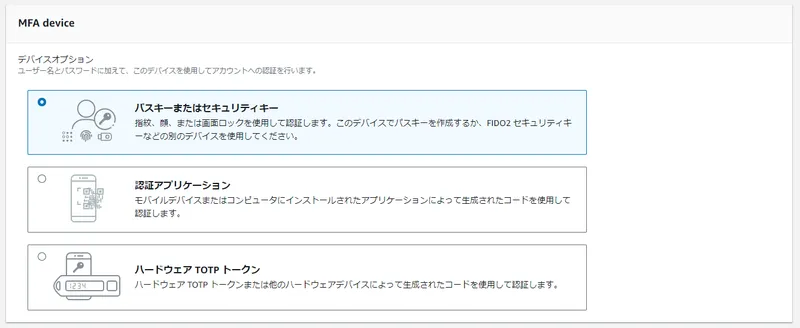
AWS IAMでPasskeyが利用可能に Windows PCで指紋認証を設定してみました
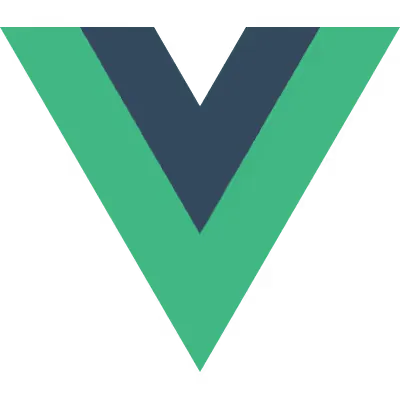
Vueのスコープ付きCSSで子コンポーネントにCSSを適用する【Vue3】
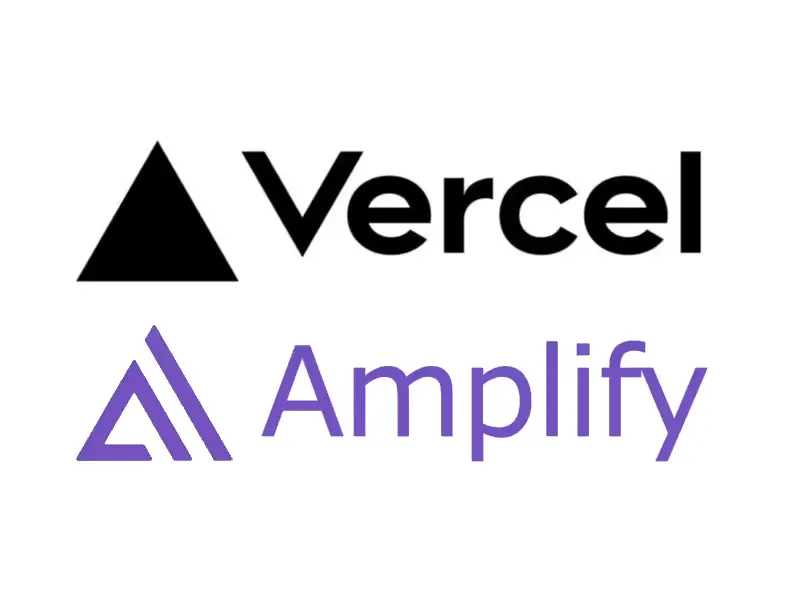
Vercel(Hobby)→AWS Amplify 移行して分かった両者の違い
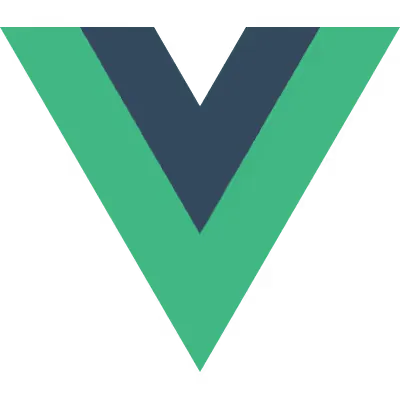
手軽に使えるVueプレイグラウンド環境。Vue SFC Playground解説
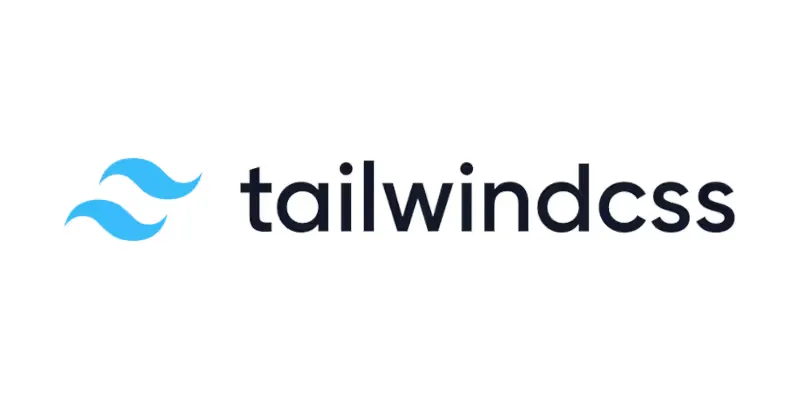
Vite、TailwindCSS v4環境を構築【Tailwind CSS v4-alpha】
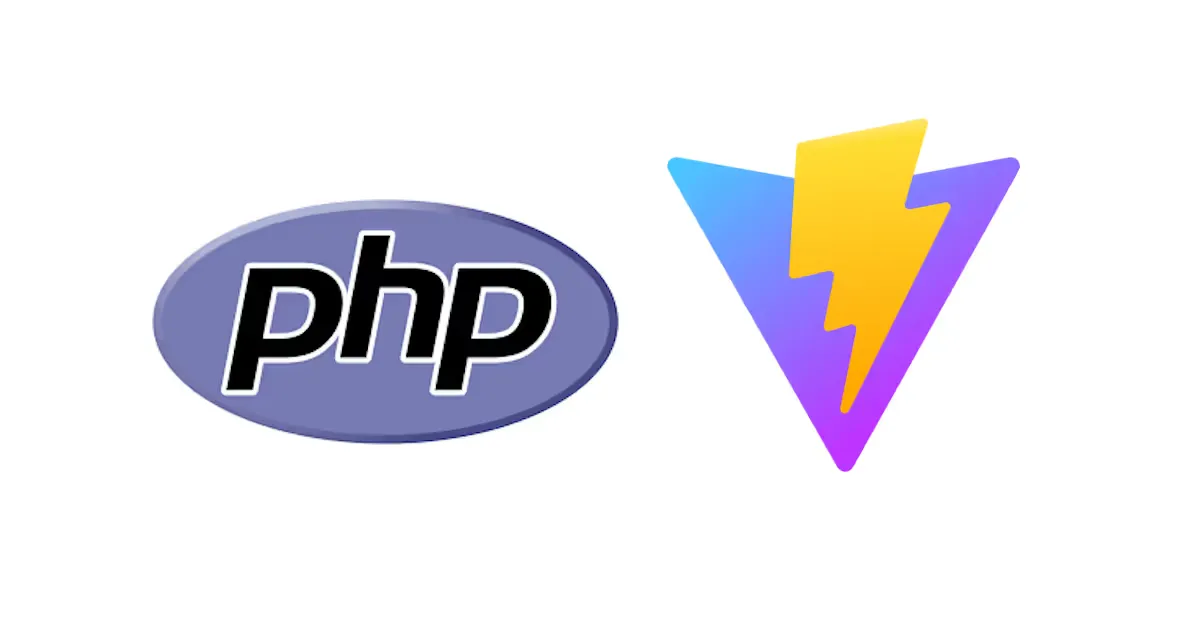
「vite-plugin-react can't detect preamble.」エラー対応【PHP、React】
オススメ記事
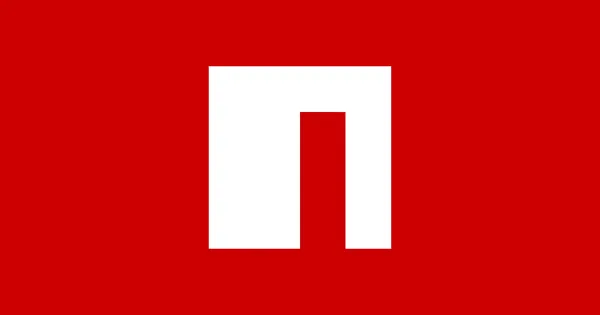
フロントエンド開発体験を向上させる10のnpmライブラリ【2024年版】
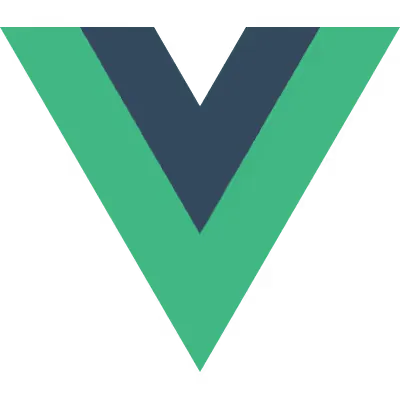
VueのdefineModelを活用した入力コンポーネントの作成方法【Vue3.4】
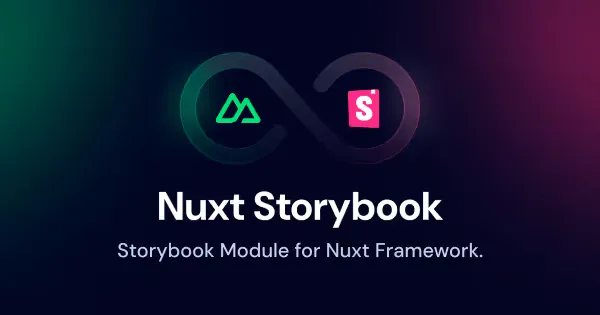
Nuxt3にStorybookを導入する方法
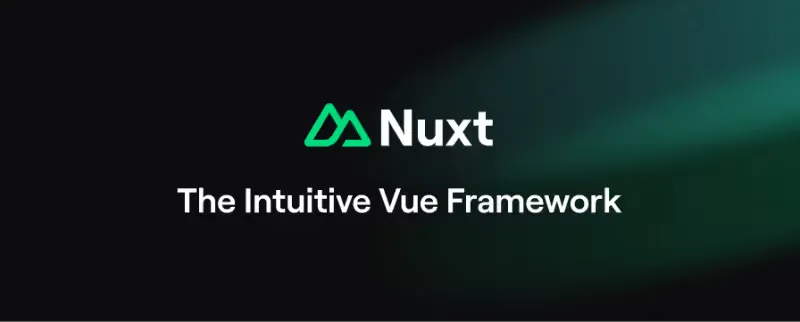
NuxtJSを使ってブログを作ってみた【Nuxt Content/TailwindCSS/daisyUI】
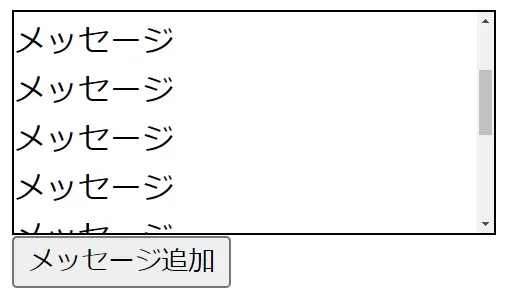